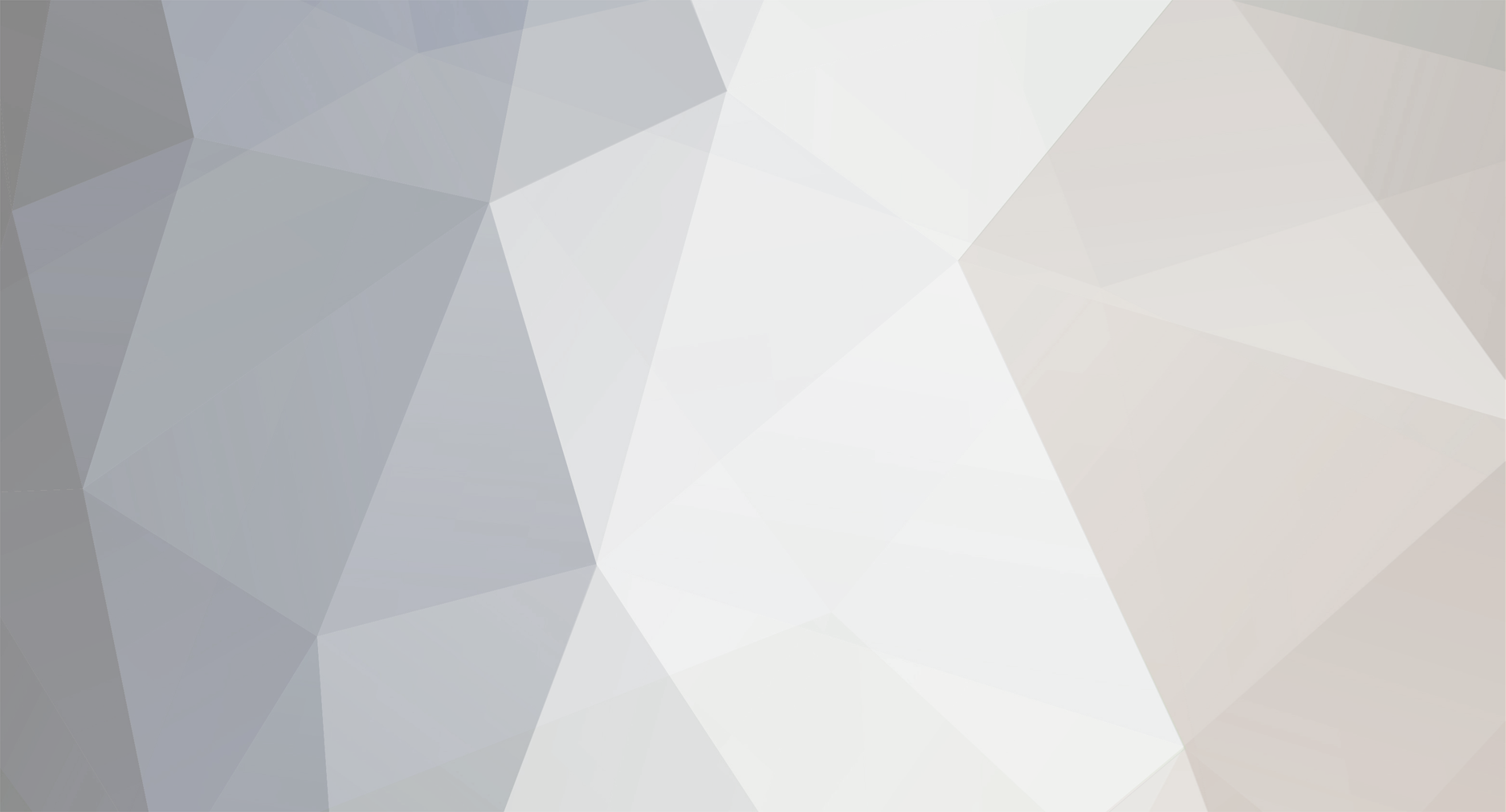
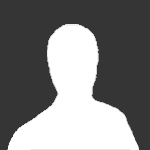
olive47
-
Posts
11 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Everything posted by olive47
-
/*
Tell DCS-BIOS to use a serial connection and use interrupt-driven
communication. The main program will be interrupted to prioritize
processing incoming data.
TACAN with 7 seg Display and TM1637
This sketch sets the Pro Mini as a Slave to the RS-485 Bus for the right console.
NOTE: #defines are to be placed before the #includes
*/#define DCSBIOS_IRQ_SERIAL
#include "DcsBios.h"
#include <TM1637TinyDisplay.h>
#include <LedControl.h>String TACAN = ""; // Declare global new string variable
char Digit0 = ""; // Declare global new character variable
char Digit1 = "";// Declare global new character variable
char Digit2 = "";// Declare global new character variable
char Digit3 = "";// Declare global new character variable
int TACAN_power = 0;int Tacan_ingame1; // hold value from DCS for ones
int Tacan_ingame10; // hold value from DCS for tens
int Tacan_ingame100; // hold value from DCS for hundreds
//FIRST - 4 DIGIT 7 SEGMENT DISPLAY- tacan
// Module connection pins (Digital Pins)
#define CLK 2
#define DIO 3
TM1637TinyDisplay display (CLK, DIO); //set up the 4-Digit Display
DcsBios::Switch2Pos tacanTestBtn("TACAN_TEST_BTN", 23);
DcsBios::LED tacanTest(0x10da, 0x0400, 13);
DcsBios::PotentiometerEWMA<5, 128, 5> tacanVol("TACAN_VOL", A0);
const byte tacanModePins[5] = {4, 5, 6, 7, 8};
DcsBios::SwitchMultiPos tacanMode("TACAN_MODE", tacanModePins, 5);
DcsBios::Switch2Pos tacanXy("TACAN_XY", 22);
DcsBios::RotaryEncoder tacan10("TACAN_10", "DEC", "INC", 9, 10);
DcsBios::RotaryEncoder tacan1("TACAN_1", "DEC", "INC", 11, 12);
DcsBios::Switch3Pos a102HmcsPw("A102_HMCS_PW", 52, 53);void onTacan10Change(unsigned int newValue) {
display.showNumber(newValue, true,2, 0);
}
DcsBios::IntegerBuffer tacan10Buffer(0x1158, 0x0f00, 8, onTacan10Change);
void onTacan1Change(unsigned int newValue) {
display.showNumber(newValue, false,1, 2);
}
DcsBios::IntegerBuffer tacan1Buffer(0x1158, 0xf000, 12, onTacan1Change);void onTacanXyChange(unsigned int newValue) {
display.showString (newValue ,1, 3); // affiche la partie chaîne en seulement le 4ème chiffre
}
DcsBios::IntegerBuffer tacanXyBuffer(0x1168, 0x000f, 1, onTacanXyChange);
// Canal TACAN
void onTacanChannelChange (char * newValue) {
Digit0 = TACAN.charAt (0); // récupère une valeur de caractère individuelle à cet index spécifique dans la chaîne
Digit1 = TACAN.charAt (1);
Digit2 = TACAN.charAt (2);
Digit3 = TACAN.charAt (3);
if (TACAN_power == 0)
display.showString(newValue, 4, 0);
}
DcsBios :: StringBuffer <4> tacanChannelBuffer (0x1162, onTacanChannelChange);
void onTacanModeChange(unsigned int newValue) {
if (newValue == 0){
display.setBrightness(0, false);}
else {display.setBrightness(15, true);}
}
DcsBios::IntegerBuffer tacanModeBuffer(0x1168, 0x000e, 1, onTacanModeChange);
DcsBios::ActionButton tacanXyToggle("TACAN_XY", "TOGGLE", 22);void setup(){
display.clear();
DcsBios::setup();
}
void loop()
{
DcsBios::loop();
}
-
ILS
/*
Tell DCS-BIOS to use a serial connection and use interrupt-driven
communication. The main program will be interrupted to prioritize
processing incoming data.
This should work on any Arduino that has an ATMega328 controller
(Uno, Pro Mini, many others).
*/
#define DCSBIOS_IRQ_SERIAL
#include "DcsBios.h"
#include "TM1637TinyDisplay6.h"
#include "DcsBios.h"
#include <LedControl.h>//FIRST - 6 DIGIT 7 SEGMENT DISPLAY-Ils
// Module connection pins (Digital Pins)
#define CLK 2
#define DIO 3
TM1637TinyDisplay6 Ils(CLK, DIO); // 6-Digit Display Class IlsDcsBios::PotentiometerEWMA<5, 128, 5> ilsVol("ILS_VOL", A0);
DcsBios::Switch2Pos ilsPwr("ILS_PWR", 4);
DcsBios::RotaryEncoder ilsMhz("ILS_MHZ", "DEC", "INC", 6, 7);
DcsBios::RotaryEncoder ilsKhz("ILS_KHZ", "DEC", "INC", 11, 12);
DcsBios::Switch3Pos a102HmcsPw("A102_HMCS_PW", 8, 9);
//ILS
void onIlsMhzChange(char* newValue) {
Ils.showString(newValue, 3, 0);
}
DcsBios::StringBuffer<3> ilsMhzStrBuffer(0x116e, onIlsMhzChange);
void onIlsKhzChange(char* newValue) {
Ils.showString(newValue, 2, 4);
}
DcsBios::StringBuffer<2> ilsKhzStrBuffer(0x1172, onIlsKhzChange);
// Canal Ils
void onIlsFrequencySChange(char* newValue) {
Ils.showString (newValue, 0, 6);
}
DcsBios::StringBuffer<6> ilsFrequencySBuffer(0x12d8, onIlsFrequencySChange);
void onIlsPwrChange(unsigned int newValue) {
if (newValue > 0){
Ils.setBrightness(15, true);
}
else {Ils.setBrightness(15, false);
}
}
DcsBios::IntegerBuffer ilsPwrBuffer(0x1168, 0x0010, 4, onIlsPwrChange);
void setup()
{
DcsBios::setup();
Ils.clear();
}
void loop()
{
DcsBios::loop();
}