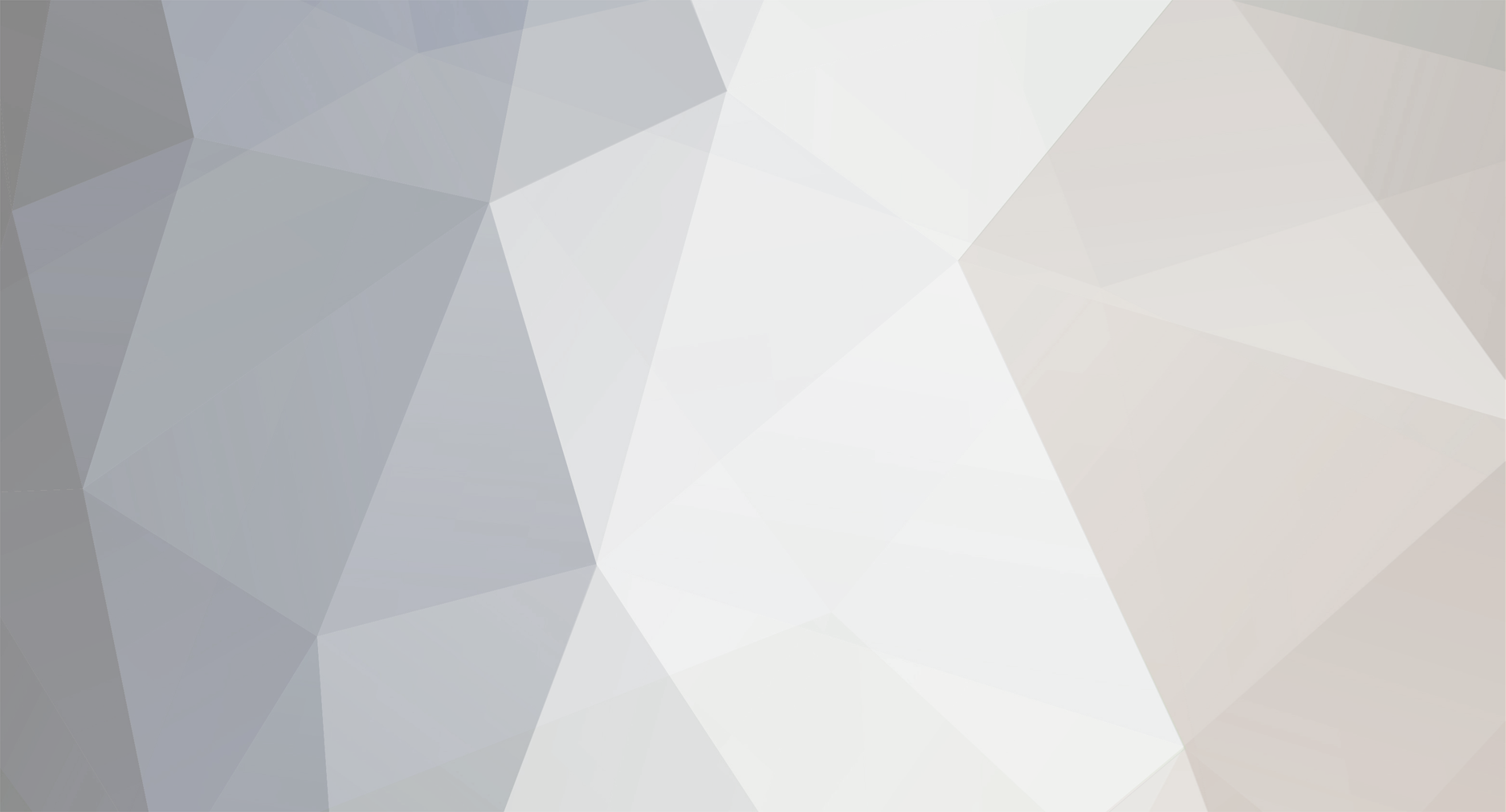
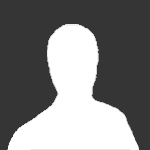
FroznAK
Members-
Posts
16 -
Joined
-
Last visited
About FroznAK
- Birthday 05/14/1980
Personal Information
-
Flight Simulators
DCS, MSFS2020
-
Location
Anchorage, AK
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
-
Wow, this is great. Thank you so much! I have some more hardware testing to do before I can try it. I wanted to confirm it was possible before getting the soldering iron out. My intent is to use this with the A-10C II module, but in theory it could be used with any TACAN control. I'm going to use a STM32 board and FreeJoy and bind the rotaries as axis-to-buttons. Still proof of concept, but I think it will be doable. Edit to add: Looking through the default.lua files in DCS, it doesn't appear that the A-10 has absolute value commands available for the TACAN control. I may not be looking in the right place. Might have to change plans and use a Pro Mirco board and the joystick.h library to do what I want.
-
I haven't needed to use the Input Command Injector Mod yet, but I've looked through various threads I could find and the Github documentation and can't find what I'm looking to do yet. Here's my specific use case: I have a TACAN control panel that adjusts the 10's and 1's channels through rotary switches. DCS only has commands for increase/decrease for these controls. What I'd like to do is have separate command inputs for each position of the rotary. Which would be 13 buttons for the 10's and 10 for the 1's. I can program clockwise and counter-clockwise rotations of the rotary to register as increase/decrease button presses, but I want the TACAN mechanical channel display to match what is in-game. I can sync my panel with the game by running a full rotation of the dials, but it would be more convenient if I didn't have to do that every time.
-
using six switches to control 8 inputs - can it be done?
FroznAK replied to lesthegrngo's topic in Home Cockpits
If you use a Pro Micro board and the Joystick.h library, you could possibly write a sketch that map multiple physical inputs to a single joystick output. A Pro Micro is recognized as a HID device and would act like the Bodnar. Alternatively, you could use a SMT32 board and FreeJoy Github and use one button as a switch register. FreeJoy is super simple to use, and boards are cheaper than the Pro Mircos. -
FroznAK started following HMA's cockpit build , Digital-To-Synchro converter for interfacing real aircraft indicators , A-10c CDU Tabletop Build and 3 others
-
Appreciate the reply and video. That's actually what I'm attempting to do here. I left out the map function coding for brevity. What I posted is trying to convert the map function result to a joystick button output. It gets complicated because the Preset switch has 20 FIXED combinations, based on the combined output from 2 rotaries. They are mechanically linked together. I think what I have will work, it's just not very elegant.
-
As for coding, using the Joystick.h library, this is what I got working for my test. There's got to be a better way to write this out, instead of essentially repeating the same lines of code over and over, and only changing the button that matches the analog input state. Any suggestions? // Test for ARC-164 Preset Channel Rotary Switch // Include the necessary libraries #include <Arduino.h> #include <Joystick.h> // include Joystick library // define Joystick Joystick_ Joystick(JOYSTICK_DEFAULT_REPORT_ID, // HID report ID, default is 0x03 JOYSTICK_TYPE_GAMEPAD, // Joystick type defaults to JOYSTICK_TYPE_JOYSTICK = joystick, JOYSTICK_TYPE_GAMEPAD = gamepad, JOYSTICK_TYPE_MULTI_AXIS = multi axis controller 10, // default 32, number of buttons 0, // number of hat switches, default 2 false, // X axis false, // Y axis false, // Z axis false, // Rx axis false, // Ry axis false, // Rx axis false, // rudder axis false, // throttle axis false, // Accelerator axis false, // brake axis false); // stearing axis // Define the pin numbers for the rotary switches // UHF Preset Channel Rotary switch consists of single dial that mechanically operates 2 rotary switches // the 1's dial has 10-positions and each position will be wired in series, separated by 1K ohm resistors and connected to a single analog input const int Preset01sPin = A0; // 10-position rotary switch (analog input) // the 10's position has 3 positions (0-2), but will only use 2 digital inputs and program logic to control off state // for test purposes only use 1 pin for 2 states (on/off) const int Preset10sPin1 = 2; // 3-position rotary switch (digital input 10's) // Variables to store the current state of the switches int Preset01sSwitchValue = 0; void setup() { // Initialize the serial communication Serial.begin(9600); // Set the pin mode for the digital switch pinMode(Preset10sPin1, INPUT_PULLUP); pinMode(A0, INPUT); // Initialize Joystick Library Joystick.begin(); } void loop() { int Preset10sSwitchState = digitalRead(Preset10sPin1); // Read the current value of the analog switch Preset01sSwitchValue = analogRead(Preset01sPin); int mappedPreset01sSwitchValue = 0; if (Preset01sSwitchValue > 0) {mappedPreset01sSwitchValue = 0;} if (Preset01sSwitchValue > 245) {mappedPreset01sSwitchValue = 1;} if (Preset01sSwitchValue > 490) {mappedPreset01sSwitchValue = 2;} if (Preset01sSwitchValue > 750) {mappedPreset01sSwitchValue = 3;} if (Preset01sSwitchValue > 1010) {mappedPreset01sSwitchValue = 4;} // Map the analog value to a range of 0 to 9 // int mappedPreset01sSwitchValue = map(Preset01sSwitchValue, 0, 1023, 0, 4); // Read the current state of the digital switch if ((digitalRead(Preset10sPin1) == 0) && (mappedPreset01sSwitchValue == 0)) { Joystick.setButton(0, 1); } else { Joystick.setButton(0, 0); } if ((digitalRead(Preset10sPin1) == 0) && (mappedPreset01sSwitchValue == 1)) { Joystick.setButton(1, 1); } else { Joystick.setButton(1, 0); } if ((digitalRead(Preset10sPin1) == 0) && (mappedPreset01sSwitchValue == 2)) { Joystick.setButton(2, 1); } else { Joystick.setButton(2, 0); } if ((digitalRead(Preset10sPin1) == 0) && (mappedPreset01sSwitchValue ==3)) { Joystick.setButton(3, HIGH); } else { Joystick.setButton(3, LOW); } if ((digitalRead(Preset10sPin1) == 0) && (mappedPreset01sSwitchValue == 4)) { Joystick.setButton(4, HIGH); } else { Joystick.setButton(4, LOW); } if ((digitalRead(Preset10sPin1) == 1) && (mappedPreset01sSwitchValue == 0)) { Joystick.setButton(5, HIGH); } else { Joystick.setButton(5, LOW); } if ((digitalRead(Preset10sPin1) == 1) && (mappedPreset01sSwitchValue == 1)) { Joystick.setButton(6, HIGH); } else { Joystick.setButton(6, LOW); } if ((digitalRead(Preset10sPin1) == 1) && (mappedPreset01sSwitchValue == 2)) { Joystick.setButton(7, HIGH); } else { Joystick.setButton(7, LOW); } if ((digitalRead(Preset10sPin1) == 1) && (mappedPreset01sSwitchValue == 3)) { Joystick.setButton(8, HIGH); } else { Joystick.setButton(8, LOW); } if ((digitalRead(Preset10sPin1) == 1) && (mappedPreset01sSwitchValue == 4)) { Joystick.setButton(9, HIGH); } else { Joystick.setButton(9, LOW); } // Add a delay to avoid rapid changes in the output delay(100); Serial.print("Preset01sSwitchValue = "); Serial.print(Preset01sSwitchValue); Serial.print("; Preset10sSwitchState = "); Serial.print(Preset10sSwitchState); Serial.print("; mappedPreset01sSwitchValue = "); Serial.println(mappedPreset01sSwitchValue); delay(100); } EDIT: updated code for syntax and completeness.
-
I acquired an authentic ARC-164(V) switching unit and decided to attempt to make it work with DCS. I'm am very new to the whole panel making and just finished my first A-10 panels (AAP, TACAN, ILS, Ext. Lighting) using PCFlights panels and Arduino's, using DCS-BIOS. It all works well, but I'd like to use the ARC-164 for multiple aircraft. My plan for the ARC-164 is to use an Arduino Pro Micro (clone) as to use it as a standard HID controller. In theory, I can use 9 analog inputs for all of the rotary switches, but my programing skills leave a lot to be desired. There are few approaches that I can take here, but I wanted to see what everyone thought would be best. I started by disassembling and cleaning the panel and working on the preset switch. This one is interesting, as it's actually 2 switches in 1. The left switch (looking from rear of panel) is for the 1st digit (0-9) and the right switch controls the 2nd digit (0-2). My plan is to wire the left switch positions into series on a pbc, with resistors between, to wire to an analog input. My question is: is there a way to also wire the right switch into series with the left, so I can use 1 input? I'm not even sure how that would work, but I have in my head it would involve a transistor or something. Or would it just be easier to wire the right switch to 1 or 2 digital inputs and program an if/else statement to control the switch state? Please keep in mind this is all theoretical in my head and I have no idea how to practically make this all work! At least I was smart enough to be able map the switches to their corresponding pins on the back of the panel on my own. lol
-
Mission 5 Additional task issues - SPOILER -
FroznAK replied to SPAS79's topic in A-10C Operation Persian Freedom Campaign
Never mind, I located the correct building. I didn’t realize there are 2 burned out BDRMs and I couldn’t see the correct one when I was orbiting to the east of the city. 2nd run through i orbited northwest of the city and it was pretty obvious which it was. Even could see the 2 “chimneys”. Just 2 little circles on the rectangular structure on top of the building. -
Mission 5 Additional task issues - SPOILER -
FroznAK replied to SPAS79's topic in A-10C Operation Persian Freedom Campaign
Anyone have a screenshot of the proper building? I see the bdrm and trucks on the street between the buildings? I hit what I thought was correct building, but obviously wasn’t. Was so disappointing, because it was otherwise a perfect run lol. Great campaign so far. So immersive. One comment, can we replace the CBU’s with just about anything else lol…they are pretty worthless. -
This is probably my favorite campaign and I have played through it a couple times now. I decided to do it again after the 2.8 cloud updates and what a difference. Weather plays a big part in these missions and the new clouds in VR add a whole new depth to the experience. Can't wait for new releases from Reflected!
-
investigating tacan issue Mission 6 Experiences
FroznAK replied to Ready's topic in A-10C Iron Flag Part I Campaign
I just finished the campaign (only took me a year to get around to it lol). I can confirm that the TACAN doesn't work in A/A, but does work in A/G mode. Loved the campaign btw. When is part 2 coming out? I can only assume it will go deeper into weapon deployment, which I sorely need! lol -
I don’t think I have a Viacom plug-in installed. I use Voice Attack through Steam. Are those the same or 2 different things. It’s been so long since I set it up.
-
Also, what batteries are we talking about here? AAA or AA? Where to they go and what are they for? New e-kneeboard?
-
I updated to 2.8 and now all my kneeboard maps are messed up. The maps are replaced with this weird image, which I have no idea what it's from. You can see the mission track lines, waypoints, and position markers on top of the image. All the other kneeboard images like checklists, mission details, ect. work fine. It's only the maps that are affected. Any idea where this image might be pulling in from?
-
investigating tacan issue Mission 6 Experiences
FroznAK replied to Ready's topic in A-10C Iron Flag Part I Campaign
I don't have a problem with the tanker responding, but I don't get any HSI response when I tune the Tacan to 10X and in A/A mode in either REC or T/R. Is this related to the bug you referred to before, or am I doing something wrong? TCN is selected on the NMSP. I end up having to use the F10 map to find the tanker, which isn't a big issue. For the actual AAR part...that's a me problem