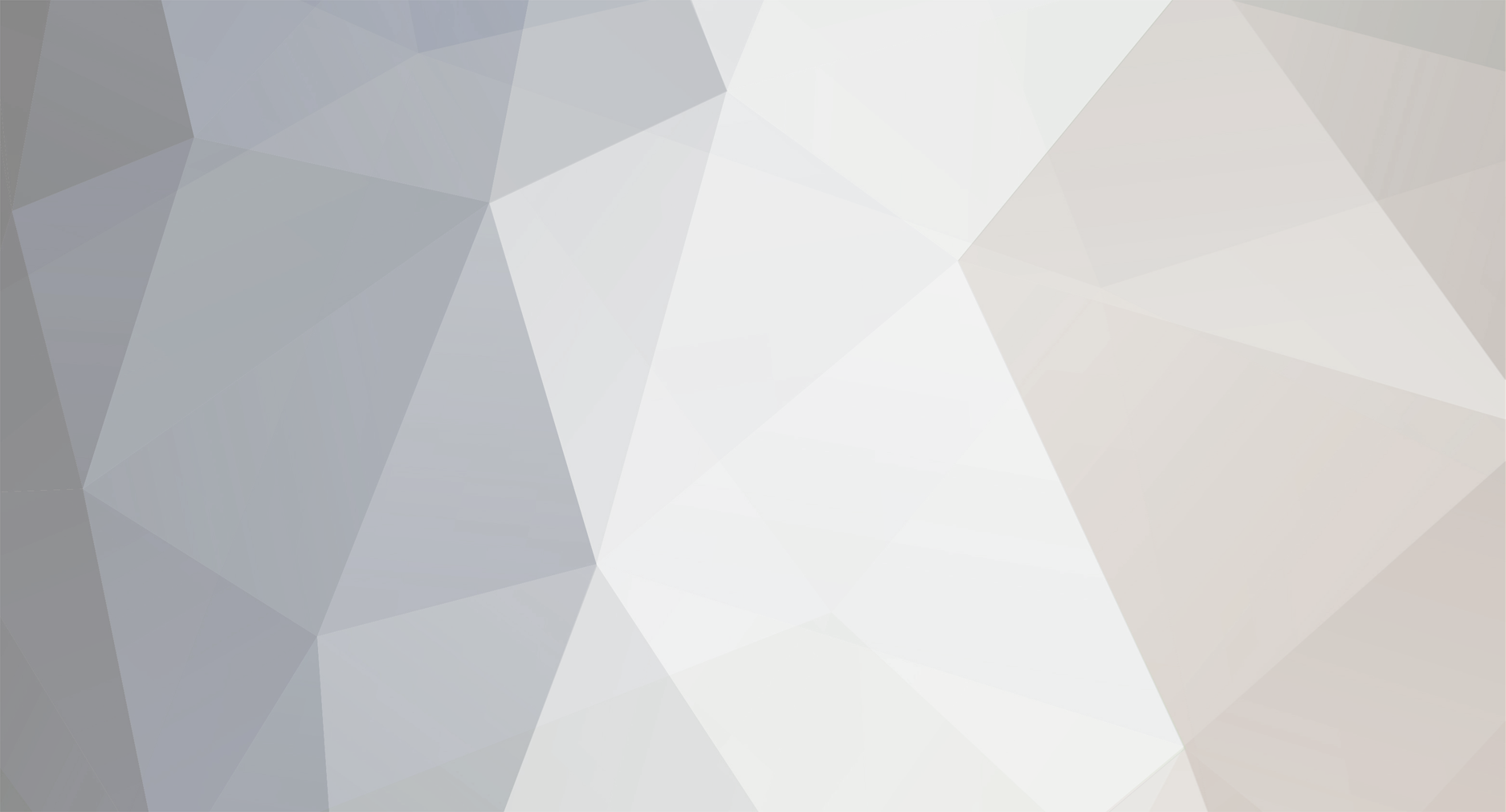
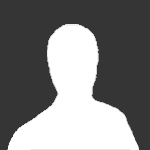
FSFIan
Members-
Posts
1301 -
Joined
-
Last visited
-
Days Won
7
Content Type
Profiles
Forums
Events
Everything posted by FSFIan
-
Just a heads-up: to play nice with other applications that use Export.lua, you should store a reference to any existing callbacks before you redefine them and make sure to call them from your own callbacks. See the Lua code for Helios or DCS-BIOS for an example. For the same reason, you must avoid global variables as much as possible. In DCS-BIOS, I put everything into a single global table named BIOS or into file-local variables. This doesn't really matter as long as you only use it internally, but once you publish anything, you will have users that want to use it concurrently with other programs such as Helios or TARS.
-
I have pushed the current state of things to the rs485 branch on GitHub. If you don't want to mess around with git, you can download a ZIP file of the contents of that branch here: https://github.com/dcs-bios/dcs-bios-arduino-library/archive/rs485.zip Here's a very quick overview of changes you need to be aware of: The DCS-BIOS Arduino library is now configured with #defines before including DcsBios.h. See the example sketches to see how this is done. onDcsBiosWrite() is gone. If you want to process an integer value, use the IntegerBuffer class like this: void onAltChange(unsigned int alt) { lcd.setCursor(0, 0); lcd.print(alt); lcd.print(" "); } DcsBios::IntegerBuffer altBuf(0x0408, 0xffff, 0, onAltChange); The template sketch has gotten much smaller, the communication code has moved into the library. This also means that once you have programmed and tested a sketch using a serial connection, you can quickly switch it to work as a RS-485 slave device by modifying the configuration #defines (see example sketches). I have quickly tested Switch2Pos, LED, StringBuffer and IntegerBuffer. Everything else could still be totally broken. Quick RS-485 How To: Flash the RS-485 Master example sketch to an Arduino Mega. Connections on the Mega (MAX487 is the transceiver chip I use): TX1 ---- DI on first MAX487 RX1 ---- RO on first MAX487 2 ---- /RE and DE on first MAX487 TX2 ---- DI on secondMAX487 RX2 ---- RO on secondMAX487 3 ---- /RE and DE on secondMAX487 TX3 ---- DI on third MAX487 RX3 ---- RO on third MAX487 4 ---- /RE and DE on third MAX487 If you do not connect a second or third transceiver, comment out the corresponding #define UARTn_TXENABLE_PIN line in the sketch. I think that up to two transceivers on the Mega should work without problems, I am not sure about three yet. For the slave device, start with the RS485Slave example sketch. Connections: TX ---- DI on MAX487 RX ---- RO on MAX487 2 ---- /RE and DE on MAX487 Between all transceiver chips, daisy-chain GND, A and B pins. Run the connect-to-serial.cmd script with the Mega's COM port.
-
The key is to either connect the Arduino's RST pin to the DTR signal from the serial adapter or to manually tap the reset button right after the Arduino IDE status switches from "Compiling..." to "Uploading...". You also have to make sure the connections are correct. Normally, you would expect the following: TX (Adapter) -- RX (Arduino) RX (Adapter) -- TX (Arduino) DTR (Adapter) -- RST (Arduino) Some adapters have RX and TX labelled the other way around, so you need to connect signals with the same name (those also tend to label the DTR signal "RST"): RX (Adapter) -- RX (Arduino) TX (Adapter) -- TX (Arduino) RST (Adapter) -- RST (Arduino)
-
You can add DCS-BIOS support for a FC3 aircraft, but it will be very limited compared to something with a clickable cockpit. For output, you can export general information (airspeed, heading, altitude ASL and AGL, pitch, bank, roll); some of that is already taken care of for all aircraft by the CommonData module. Depending on the aircraft, you might have access to a little additional state, e.g. whether the autopilot is on or off. What you don't get are the positions of switches and gauge needles or the state of indicator lights, because there is no known way to grab cockpit arguments from a FC3 aircraft. For the input direction, DCS-BIOS (i.e. Export.lua) probably won't be able to do anything that doesn't also have a keybinding in the DCS options. This means that if all there is is a "toggle this switch" command, you won't be able to use Lua/DCS-BIOS to properly make a toggle switch work either. See the attachment of this post for a list of all commands you can send to a FC3 aircraft (not all of those commands apply to all airframes).
-
Almost -- I am going for an RS-485 bus with Arduino Pro Minis, which are very similar to Nanos. The Pro Mini has two analog input pins less than the Nano, does not have a USB adapter on board, and it is slightly smaller and cheaper because you don't have to pay for a onboard USB-to-serial chip that won't see any use once the code works and the board is connected to the RS-485 bus. But since the Nanos use the same ATMega328 controller, they will be just as well supported as Pro Mini boards, and at the prices below the decision between the Pro Mini and the Nano is not one driven by price -- just use whichever is more convenient for a particular panel. On AliExpress, I can find: Arduino Pro Mini for 1.36 € (separate USB adapter: 55 cents) Arduino Nano for 1.50 € Arduino Mega (without USB cable) for 5.69 € (On eBay, the price difference between the Nano and Pro Mini is a little more, about 0.64 €.) I plan to also support the Mega as an RS-485 slave device, but before I can do that I need to order a second Mega :) Let's do a quick comparison between one Mega and four Pro Minis, assuming we need three pins (RX, TX, TX_ENABLE) to connect them to a RS-485 transceiver. Arduino Mega 2560 Usable GPIO Pins: 67 RAM: 8K Program Flash: 256K Cost including one RS-485 transceiver: about 5.90 € 4 Arduino Pro Minis Usable GPIO Pins: 60 (15 each), 67 if you count A6 and A7 which are analog input only RAM: 2K each Program Flash: 32K each Cost including four RS-485 transceivers: about 6.24 € In my opinion, unless you have special requirements (e.g. need lots of flash to store images/fonts for a graphical display), four Pro Minis are better than one Mega: More processing power in total (the Mega runs at 16 MHz just like the Pro Mini). I honestly have no idea whether a Mega with lots of outputs would even work reliably. Each panel can get its own microcontroller and can be built, tested and maintained independently Helps to avoid Kabelsalat because you don't have to route 70 wires to one board In general, I would stay away from Arduino(-compatible) boards that are not powered by either the ATMega328 or the ATMega2560 controller. DCS-BIOS will probably work with them, but only in a "compatibility mode" using the default Serial library. That means no RS-485 support and no properly interrupt-driven serial communications. They can still be a good choice for special cases, such as running a CDU display on some ARM-based board running at 80 MHz.
-
A quick update: I think I have most of the features I wrote about half a year ago working now (everything except the shift register and I/O expander support). Before this can become an official release, I need to check and update all of the relevant documentation and do some minor modifications to the auto-generated code examples. Given my current university workload, that will probably take a while. In the meantime, I plan to put some minimal documentation together (a short document listing the changes compared to the current version, a few instructions on how to use the RS-485 stuff, maybe a short video) and then release the current state of things as a beta version. Assuming the headaches stop after Monday's dentist appointment, you can probably expect an update sometime next week.
-
I don't think you will get it to work without modifying the files in the DCS installation directory. I think that DCS does the following (I don't know for sure, but it would be the straightforward way to do it and be consistent with everything I have read about this): Read the list of possible key bindings from mods\aircraft\a-10c\input\... Read the list of user key bindings from the .diff.lua file For each possible key binding: Build the key it would have in .diff.lua. If there is a .diff.lua entry for it, change the key binding to the one from the .diff.lua file. Note that this means that an entry in .diff.lua will be ignored unless there is a corresponding entry in the file that defines the possible key assignments, which is located in the DCS installation directory.
-
I have given this some more thought and think it will be best to deal with this feature as part of DCS-BIOS 2.0, where I plan to change a bunch of things under the hood. If I were to implement this now, I'd only end up rewriting the code in a few weeks.
-
If you want non-intrusive, how about using panels that have no function in the sim (e.g. in the A-10C: IFF, encrypted radio, video recorder, TISL, blank buttons on the UFC) or ones that are functional but you never end up using (circuit breakers)? Another way to hide push buttons is to use rotary encoders with a push button in places where the sim has just a rotary, e.g. the HSI heading and course adjustments. You can also use a modifier (e.g. paddle button on the TM Warthog stick) to map additional things to your HOTAS. The TM Warthog throttle also has a button that to my knowledge does not have a function in the real aircraft: pushing the mic switch in towards the throttle lever :) Or be sneaky and make one of your MFCDs a touchscreen where a menu pops up when you tap the screen. If you use a Logitech G930 wireless headset, that also has three buttons that you can program to do whatever you want. For emergencies (sim freezes and needs to be killed), hide a wireless keyboard with an integrated trackball under the seat.
-
There is no easy way to do this right now -- the DCS-BIOS Arduino Library is written so that input is only sent to DCS when a switch changes state. This was a deliberate decision because in some situations, doing this can be dangerous or annoying (e.g. switching off the battery and generators in a hot start or air start mission). And even in a cold start scenario, wouldn't you want to do it the other way around (switch your physical switches to the state they are in your virtual aircraft) so you can properly execute your start-up checklist? If there is actually a use case for this, I think I could write a function that you could call on the Arduino side that makes all of the inputs send their current state to DCS. You could then call that in the setup() function and whenever the current aircraft name changes, so it would be executed whenever you hop into a new aircraft. But before I spend time on that, I'd like to hear some reasons why you would want to have automatic control synchronization. It's not that I am against implementing it, but I never saw the point in the "synchronize controls on startup" option in DCS either (too many times have my engines shut down after joining a hot start or air start A-10C when I had that on), and I don't want to feel like I am implementing a useless feature :)
-
Released v0.4.2. A-10C: Add SET_VHF_AM and SET_VHF_FM commands to directly set the radio frequencies for the AM and FM radios. Only works for valid frequencies for the given radio. A-10C: add ALT_PNEU_FLAG export value (position of the yellow PNEU flag in the Altimeter) Please post comments in the DCS-BIOS Discussion Thread.
-
It should work with any Arduino, so give it a try if you already have the Due, but I'd recommend ATMega328-based boards (Uno, Pro Mini, ...) and possibly the Mega 2560. I test my code on Pro Mini boards and some future improvements for the Arduino library (interrupt-based communications and RS-485) will only work on the ATMega328 (and maybe the Mega 2560) unless someone else ports them to other platforms.
-
Connect a PS/2 keyboard to your CDU with an Arduino and DCS-BIOS
FSFIan replied to FSFIan's topic in Home Cockpits
"Would this work?" is a question you can ask your compiler / hardware. Just write it into a sketch and find out! A better question for a web forum is "Why doesn't this work? I thought it would do X, but instead Y is happening." In this case, the button would get pushed down when you press 'q' and released when you press any other key. Modifying code without understanding how it works rarely ever works out (trust me, I speak from personal experience). Even when it does what you expect it to on the first test, there can still be lots of edge cases that don't. You need to understand what you are doing. If you don't, read documentation and tutorials until you do. When you get stuck reading documentation, ask a specific question and state where you got stuck, what you tried, and how it behaved differently from what you expected. I'd suggest to take further discussion of this project to a separate thread. -
If you follow the steps in the developer guide, you should get to the point where you have a M-2000C module in the control reference docs. Here's an M-2000C.lua to get you started: BIOS.protocol.beginModule("M-2000C", 0x2400) BIOS.protocol.setExportModuleAircrafts({"M-2000C"}) local document = BIOS.util.document local defineToggleSwitch = BIOS.util.defineToggleSwitch local defineIndicatorLight = BIOS.util.defineIndicatorLight defineToggleSwitch("BATTERY_POWER", 8, 3520, 520, "Electrical Power Panel", "Battery Power") -- caution panel light argument numbers start at 525 defineIndicatorLight("CLP_BAT", 525, "Caution Lights Panel", "BAT") defineIndicatorLight("CLP_TRW", 525, "Caution Lights Panel", "TRW") -- increasing argument numbers from left to right, top to bottom defineIndicatorLight("CLP_DSY", 551, "Caution Lights Panel", "DSY") BIOS.protocol.endModule() Note that the comments in devices.lua are misleading, you need to add 1 to each device ID (if you read through the code for the counter() function, you'll see that it starts counting from one, not from zero as the comments assume). I think that the reason that the indicator lights are nowhere to be found in the Lua files is that they do not have tooltips, so there is no reason for them to interact with the mouse pointer in any way. They still have cockpit arguments. The easiest way to find out which cockpit arguments are for which lights is probably to ask RAZBAM if they have a list of them. Otherwise, use brute force! Start with this code snippet in the Lua Console: for i=1,1000 do GetDevice(0):set_argument_value(i, 1) end When you execute that, you will see all kinds of lights flashing briefly for one frame or so before they are reset again by the M-2000C code. Now you can do a binary search -- adjust the range of the loop until the only light that is flashing is the one you want. Using that technique, I found out that the lights for the caution lights panel in the M-2000C start at argument number 525. This code snippet (and my earlier post about how to export the M-2000C radar with MonitorSetup.lua) is brought to you by the M-2000C Test Key (I would not have bought a fast mover module), so thanks again to ED for making me a beta tester! :thumbup:
-
The JSON file is just a serialization of the "documentation" property of the module object. It is rewritten automatically when DCS-BIOS is started. Look at the function BIOS.util.document and how it is being used elsewhere (e.g. in the example in the developer guide or in BIOS.util.definePushButton()). For most cases, you will use one of the functions in BIOS.util and those take care of adding the documentation entry.
-
Connect a PS/2 keyboard to your CDU with an Arduino and DCS-BIOS
FSFIan replied to FSFIan's topic in Home Cockpits
You need to explicitly set the joystick button to "off" (I don't know the Teensy libraries, but I assume that would be "Joystick.button(3, 0);"). Ideally, you want to do this when the button on the actual keyboard is released. The sketch in the original post does not address this, as it was hacked together in half a day (it simply immediately releases the button in DCS after pressing it). You need to figure out how to get separate "key down" and "key up" events out of the PS/2 keyboard library (which might require modifications to the library). It may also be easier to work directly with the scan codes that the keyboard sends instead of translating to characters first, this way you don't need special handling for shift and caps lock. In that case, the Teensy is definitely the right choice (with the possible alternative of the Arduino Pro Micro). V-USB requires extra soldering and more external components (three resistors, two diodes, USB jack) and IIRC overpro's sketch requires re-flashing the ATMega16U2 on the Uno board -- not that difficult, but more complicated than simply uploading a sketch. Your chances of success are not limited by what you know. They are only limited by how much you are willing to learn and the amount of time you can invest into this project. -
Connect a PS/2 keyboard to your CDU with an Arduino and DCS-BIOS
FSFIan replied to FSFIan's topic in Home Cockpits
If you want to make a PS/2 Keyboard to USB Game Controller adapter, I'd start with a project that turns your microcontroller into a USB Game Controller and add the PS/2 Keyboard library to that. Depending on your existing experience / willingness to learn, there are several options available, each with slightly different trade-offs between cost and effort. The cheapest option is probably an ATMega328 running V-USB. V-USB is pretty easy to get up and running in a traditional Linux development environment (any text editor, make, avr-gcc, flash with avrdude). I don't know how much work it would be to use it with AVR Studio or the Arduino IDE, as I have never tried that. Another option is to use a microcontroller with native USB support. There are Arduino boards with the ATMega32u4 (Leonardo, Pro Micro), or you could use a Teensy board. Use your favorite search engine to find a USB Game Controller code example that you can understand and work from there. -
DCS-BIOS does not export the state of any external lights. Currently, your best bet is to figure out their state based on looking at some combination of switches (battery power + light switch) and then implementing the blinking on the Arduino. Since the external lights are not displayed in the internal cockpit model, there is no cockpit argument for them (and also no other way to grab them from Export.lua). A few months ago, I discovered a roundabout way to grab the draw arguments for the external aircraft model, which to my knowledge also include the state of the external lights. I do plan to integrate that into DCS-BIOS 2.0 at some point, but that is still many, many months away.
-
Don't worry about it, component failure is evil and happens to all of us. It's fun to help people like you -- people whom I can give a few hints and suggestions, and they go back and experiment some more on their own, and a few posts later the problem is solved. That's time well spent. What annoys me are those who ask things they can look up in the first three Google hits, don't provide enough context for me to give a concise answer, and who, when pointed to other online resources, do not ask a sensible follow-up question but instead want me to explain everything from the ground up or write their sketch for them. Fortunately, those people are few and far between and given that I don't sell DCS-BIOS, I am free to ignore them :)
-
I am not an expert on analog stuff by any means, but on a 50 cm ribbon cable, with the internal pull-up resistor on the Arduino (which is somewhere between 30k and 50k), crosstalk sounds like a plausible explanation to me. You can try a "stronger" pull-up resistor. Simply connect a resistor (anything between about 1k and 10k) between the FQIS_TEST Arduino pin and +5V. If a pull-up resistor value is too high, the line is more susceptible to noise. If the pull-up resistor is too low, a large current will flow through it when the button is pressed, which increases power consumption (important in battery-powered applications) and in extreme cases (less than 125 ohm) will exceed the current limit of 40 mA per pin on your microcontroller. If that doesn't help, double-check your wiring and then post a link to the full sketch and a schematic (a photo will do as long as it's clearly apparent where the wires go).
-
If you want to add a debouncing delay, I'd recommend adding it in your loop() function. Are you sure that your problem is caused by bouncing signal lines? (Does it send multiple lines of text to DCS when you move the encoder by a single detent?) The RotaryEncoder class in DCS-BIOS requires it to go through all four states in the right order before it recognizes a step, it should be fairly robust against bouncing already. Do you have a datasheet for the rotary encoders? (Maybe they do something weird like send eight pulses per step.) Can you describe the "strange jump" in more detail? Does it also happen if you send the command through the interactive control reference documentation? (If it does, it's definitely not your Arduino code.)
-
Der Throttle vom TM Warthog steht neben meinem Stuhl auf einem alten PC-Gehäuse und hat damit genau die richtige Höhe. Für Stick und Pedale hab ich mir eine Holzkonstruktion gebaut, wo ich auch den Bürostuhl mit einem kurzen Spanngurt dran festbinde, damit der nicht wegrollt. Das Ding will ich mir aber bei Gelegenheit mal aus leichteren Materialien neu bauen, damit ich es einfacher zur Seite schieben kann.
-
Thanks for the offers! To provide support for additional modules, help from people who actively fly the airframe in question is invaluable. Even if I had enough time to add all of them myself (which I don't), I couldn't test everything properly because I wouldn't know how it's supposed to behave (e.g. to test whether exporting a certain indicator works, you need to know what other things to do to turn it on and move it around). Let's talk about this in two or three weeks. I plan to do a bunch of work on DCS-BIOS over the Christmas break. Depending on how far I get the way to add additional aircraft may change significantly, so any knowledge obtained now could become obsolete. I have a few ideas about how to improve the debugging capabilities and automate some repetitive parts of the process.
-
Solderless PCB mounting for pushbuttons, on-off switches etc
FSFIan replied to pappavis's topic in Home Cockpits
You might also want to consider designing your shields to plug onto a Pro Mini instead, so your PCB is a lot smaller and thus cheaper to produce. Surface mount components are small and as long as you use the larger sizes (1205 or 0805 for two-lead components like resistors, and SOIC for the IC packages), they are not significantly harder to solder than through-hole stuff, especially on a professionally made PCB where you have a solder mask.